Uplift iOS Interview
The Guide is for YOU if
- You are preparing for an iOS interview and want to improve your skills and knowledge and looking to level up your interview game and land your dream job.
- You want to gain confidence and ease during iOS interviews by learning expert tips and curated strategies.
- You want access to a comprehensive list of iOS interview QA to practice and prepare.
SOLID is a set of design principles that aim to make the software more modular, maintainable, and extensible. In the context of app/software development, here is a brief overview of each principle:
- Single Responsibility Principle (SRP): A class should have only one reason to change. It should be responsible for one specific task, and it should not have multiple responsibilities.
- Open/Closed Principle (OCP): A class should be open for extension but closed for modification. This means that you should be able to add new functionality to a class without changing its existing code.
- Liskov Substitution Principle (LSP): Subtypes should be substitutable for their base types. This means that a subclass should be able to be used in place of its parent class without causing any unexpected behavior.
- Interface Segregation Principle (ISP): A client should not be forced to depend on methods it does not use. This means that interfaces should be designed to be specific to the needs of each client, and should not contain unnecessary methods.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules. Both should depend on abstractions. This means that you should program to interfaces, rather than concrete implementations, and that dependencies should be injected, rather than hard-coded.
Many people have a hard time understanding and remembering the SOLID Principles. However, I can simplify and rephrase them in a way that will make it easier for you to remember and explain them to others.
The explanation for my 10 Years Old Nephew
SOLID Principles are a set of rules that help people write really good computer programs. Just like how we need to follow some rules to build a strong and sturdy house, we need to follow some rules to build good computer programs that work well.
The SOLID Principles are named after each of the letters in the word “SOLID”, which stands for:
- S – Single Responsibility Principle: Each part of the program should only be responsible for doing one thing.
- O – Open/Closed Principle: The program should be able to add new features without changing the existing code.
- L – Liskov Substitution Principle: New parts of the program should be able to replace older parts without causing any problems.
- I – Interface Segregation Principle: Different parts of the program should only use the parts that they need, instead of everything.
- D – Dependency Inversion Principle: Different parts of the program should depend on abstractions (ideas), instead of depending on specific things.
By following these rules, we can make sure that our computer programs are easy to understand, easy to change, and work really well.
As an iOS Developer, Why should you learn SOLID principles?
As an iOS developer, learning the SOLID principles is crucial to becoming a better programmer and developing maintainable, scalable, and extensible software.
Here are a few reasons why you should learn SOLID principles as an iOS developer:
- Improving code quality: SOLID principles provide guidelines for writing clean, organized, and maintainable code. Adhering to these principles makes your code more readable, understandable, and less prone to bugs. You can write code that is easier to understand, extend, and refactor, making it easier for you to maintain and improve it over time.
- Reducing technical debt: Technical debt is the cost of maintaining code that was poorly designed or implemented. If you don’t follow SOLID principles, you may end up with code that is difficult to change or improve, leading to more technical debt. By applying SOLID principles, you can avoid creating technical debt and make your codebase more sustainable and easier to maintain over time.
- Enabling collaboration: If your code follows SOLID principles, it is easier for other developers to understand and work with your code. Code that is modular, decoupled, and easy to test allows for better collaboration between developers, leading to faster development cycles, higher quality code, and better software.
- Building scalable applications: SOLID principles allow you to build applications that are scalable and easy to maintain. By following these principles, you can design code that can grow and change over time without becoming unmanageable or causing performance issues.
- Preparing for future changes: The iOS development landscape is constantly evolving, and new features and technologies are always being introduced. SOLID principles provide a solid foundation for developing applications that can adapt to future changes. By writing modular and extensible code, you can add new features or technologies without having to rewrite significant portions of your codebase.
In summary, learning SOLID principles as an iOS developer is essential for building high-quality software that is easy to maintain, scalable, and adaptable. By adhering to these principles, you can build better code and become a better developer, making your work more enjoyable and fulfilling.
Enforcing SOLID Principles in iOS App Development
Implementing and enforcing SOLID principles in iOS app development can be challenging, but it is also critical to building high-quality, scalable software. Here are five tips for implementing and enforcing SOLID principles in iOS app development:
- Start with the basics: Start by focusing on the Single Responsibility Principle (SRP), which is the foundation of SOLID principles. Ensure that each class or module has a single, well-defined responsibility. This helps keep the code modular, easier to understand, and less prone to bugs.
- Apply the Open/Closed Principle (OCP): When writing code, strive to create modules that are open for extension but closed for modification. This means that adding new features to a module should be possible without modifying the existing code. You can achieve this by using protocols and inheritance to define abstract interfaces, rather than relying on concrete implementations.
- Use protocols to apply the Liskov Substitution Principle (LSP): When using inheritance, make sure that subclasses can be used interchangeably with their base classes. Use protocols to define abstract interfaces, and ensure that all classes that implement these interfaces can be used interchangeably.
- Separate concerns using the Interface Segregation Principle (ISP): Use protocols to define specific interfaces for each responsibility of a class or module. This helps to ensure that each module has only the methods it needs, reducing the risk of unexpected dependencies and making the code easier to test.
- Practice Dependency Inversion Principle (DIP): To achieve loosely coupled code, always depend on abstractions, not concretions. This means using protocols to define dependencies between modules, rather than relying on concrete classes. This helps to reduce dependencies and makes it easier to swap out implementations in the future.
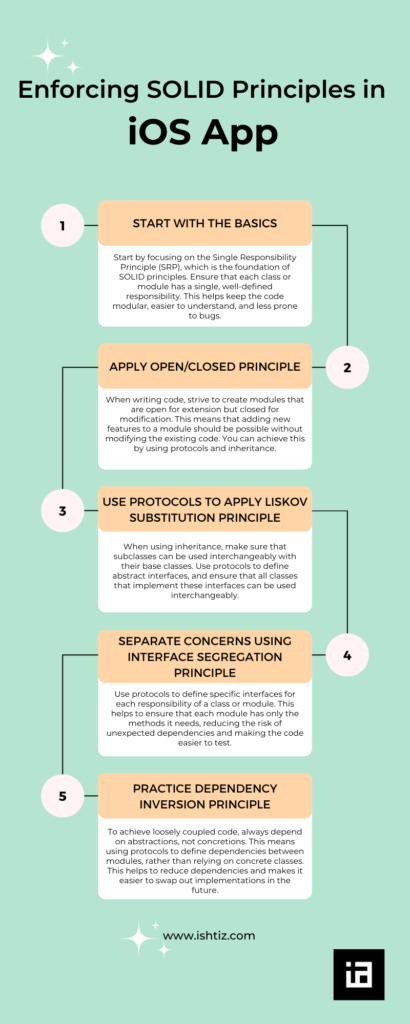
Implementing SOLID principles in iOS app development requires a deliberate approach and a willingness to invest in writing high-quality code.
Top Challenges for Implementing SOLID in iOS app
Implementing SOLID principles in iOS app development can be challenging. Here are the top three challenges that iOS developers may face when implementing SOLID principles in their apps:
- Understanding the principles: SOLID principles can be difficult to understand and apply, particularly for developers who are new to software design and architecture. Some of the principles, such as the Dependency Inversion Principle (DIP), may be particularly challenging to implement, requiring a deeper understanding of object-oriented programming concepts.
- Balancing SOLID principles with other design considerations: SOLID principles are not the only design considerations when building iOS applications. Developers may also need to consider performance, user experience, and platform-specific requirements. Sometimes, adhering to SOLID principles may lead to more complex code or reduced performance, making it challenging to find a balance between SOLID principles and other design considerations.
- Legacy code: iOS apps often have legacy codebases that may not conform to SOLID principles. Updating these codebases to adhere to SOLID principles can be time-consuming and challenging, particularly if the codebase is large or if other developers are unfamiliar with SOLID principles. It may be necessary to refactor code incrementally over time or to educate other developers about the benefits of SOLID principles.
Implementing SOLID principles in iOS app development can be challenging, particularly for developers who are new to software design and architecture. Balancing SOLID principles with other design considerations, understanding the principles, and updating legacy code can all be obstacles to implementing SOLID principles in iOS apps. However, with a deliberate approach and a willingness to invest in high-quality code, developers can overcome these challenges and build high-quality, maintainable, and scalable iOS applications.
Is SOLID contradictory with other principles in iOS?
SOLID principles are not inherently contradictory with other principles in iOS development. However, it is possible that adhering strictly to SOLID principles could create tensions with other design considerations, such as performance, user experience, or platform-specific requirements. For example, adhering to the Single Responsibility Principle (SRP) may require developers to create more classes or modules, which could negatively impact performance.
Furthermore, there may be instances where certain SOLID principles may be less applicable to specific features or components of an iOS app. For example, the Interface Segregation Principle (ISP) may be less relevant for small components or features that do not require complex interfaces.
Ultimately, implementing SOLID principles in iOS development requires a balance between adhering to the principles and addressing other design considerations. While there may be situations where SOLID principles are not the best approach, in general, following SOLID principles can help create a more maintainable and scalable codebase.
Let’s go though the principles one by one with example:
Single Responsibility Principle (SRP)
The Single Responsibility Principle (SRP) states that a class should only have one reason to change. In other words, a class should only be responsible for one thing, and not have multiple responsibilities.
Let’s take an example of an iOS app that shows a list of movies. One class, MovieListViewController, is responsible for displaying the list of movies on the screen. Another class, MovieDataProvider, is responsible for providing the list of movies to the MovieListViewController.
If we apply the SRP, we can see that MovieListViewController should not be responsible for getting the data, because its job is to show the list of movies, not to provide the data. On the other hand, MovieDataProvider should only be responsible for getting the list of movies, and not for displaying them on the screen.
By separating the responsibilities of these classes, we make our code more modular and easier to maintain. If we need to change the way we display movies, we only need to change the MovieListViewController, and if we need to change the way we get the list of movies, we only need to change the MovieDataProvider. This way, we can avoid having to change multiple parts of the code when we need to make changes.
Open/Closed Principle (OCP)
The Open/Closed Principle (OCP) states that classes should be open for extension but closed for modification. This means that we should be able to add new functionality to a class without changing its existing code.
Let’s consider an example of an iOS app that has a Payment
class that handles the process of making a payment. The app supports multiple payment methods, such as credit card, PayPal, and Apple Pay.
If we apply the OCP, we should design the Payment
class in a way that allows us to add new payment methods without having to modify its existing code. One way to achieve this is to use the Strategy pattern, which separates the behavior of the payment methods into separate classes that implement a common interface.
Here’s an example:
protocol PaymentMethod { func pay(amount: Double) } class CreditCardPayment: PaymentMethod { func pay(amount: Double) { // Process the credit card payment } } class PayPalPayment: PaymentMethod { func pay(amount: Double) { // Process the PayPal payment } } class Payment { private let paymentMethod: PaymentMethod init(paymentMethod: PaymentMethod) { self.paymentMethod = paymentMethod } func makePayment(amount: Double) { paymentMethod.pay(amount: amount) } }
In this example, the Payment
class takes a PaymentMethod
object in its constructor. This allows us to pass in any object that conforms to the PaymentMethod
protocol, without having to modify the Payment
class.
If we want to add a new payment method, such as Apple Pay, we can simply create a new class that implements the PaymentMethod
protocol, and pass it to the Payment
class. We don’t need to change the existing code in the Payment
class.
This way, our code is open for extension, because we can add new payment methods without modifying the Payment
class, but closed for modification, because we don’t need to change the existing code to add new payment methods.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle (LSP) states that subtypes should be substitutable for their base types, meaning that objects of a superclass should be able to be replaced with objects of a subclass without affecting the correctness of the program.
Let’s consider an example of an iOS app that has a Vehicle
class, and a subclass Car
. The Car
class has a startEngine()
method that starts the car’s engine.
If we apply the LSP, we should be able to use an object of the Car
class wherever an object of the Vehicle
class is expected, without affecting the correctness of the program. This means that the startEngine()
method of the Car
class should not have any additional requirements or constraints that the Vehicle
class does not have.
Here’s an example:
class Vehicle { func drive() { // Drive the vehicle } } class Car: Vehicle { func startEngine() { // Start the car's engine } override func drive() { // Drive the car } }
In this example, the Car
class is a subclass of the Vehicle
class, and overrides the drive()
method to implement the specific behavior of a car. The Car
class also has a startEngine()
method that is specific to cars.
If we create an instance of the Car
class and assign it to a variable of the Vehicle
type, we can call the drive()
method without any issues:
let vehicle: Vehicle = Car() vehicle.drive() // This will call the drive() method of the Car class
However, if we try to call the startEngine()
method on the vehicle
object, we will get a compiler error:
vehicle.startEngine() // This will generate a compiler error
This is because the startEngine()
method is specific to the Car
class, and is not part of the Vehicle
class.
In this example, we have applied the LSP by ensuring that the Car
class is substitutable for the Vehicle
class, and can be used wherever a Vehicle
object is expected, without affecting the correctness of the program.
Interface Segregation Principle (ISP)
The Interface Segregation Principle (ISP) states that clients should not be forced to depend on methods that they do not use. This means that classes should have specific interfaces for the functionality they provide, and clients should only depend on the interfaces they need.
Let’s consider an example of an iOS app that has a Messaging
protocol that provides different messaging functionalities, such as sending a text message or sending an image message.
If we apply the ISP, we should design the Messaging
protocol in a way that allows clients to depend only on the specific messaging functionalities they need, without having to implement methods they don’t use.
Here’s an example:
protocol TextMessage { func sendTextMessage() } protocol ImageMessage { func sendImageMessage() } class MessageSender: TextMessage, ImageMessage { func sendTextMessage() { // Send a text message } func sendImageMessage() { // Send an image message } }
In this example, we have defined separate protocols for the TextMessage
and ImageMessage
functionalities, and the MessageSender
class implements both protocols. This allows clients to depend on only the specific messaging functionalities they need, without having to implement methods they don’t use.
For example, if a client only needs to send text messages, they can depend on the TextMessage
protocol:
class TextMessageSender { private let messageSender: TextMessage init(messageSender: TextMessage) { self.messageSender = messageSender } func send() { messageSender.sendTextMessage() } }
In this example, the TextMessageSender
class depends only on the TextMessage
protocol, and can be initialized with any object that implements the TextMessage
protocol, including the MessageSender
class.
This way, our code follows the ISP by providing specific interfaces for the functionality they provide, and clients depend only on the interfaces they need.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle (DIP) states that high-level modules should not depend on low-level modules, but should depend on abstractions. This means that the details of the implementation should depend on abstractions, rather than the other way around.
Let’s consider an example of an iOS app that has a NetworkingService
class that handles network requests. The NetworkingService
class depends on the low-level URLSession
class to make network requests.
If we apply the DIP, we should design our code in a way that allows the high-level modules to depend on abstractions, rather than depending on low-level modules.
Here’s an example:
protocol URLSessionProtocol { func dataTask(with url: URL, completionHandler: @escaping (Data?, URLResponse?, Error?) -> Void) -> URLSessionDataTask } extension URLSession: URLSessionProtocol {} class NetworkingService { private let urlSession: URLSessionProtocol init(urlSession: URLSessionProtocol) { self.urlSession = urlSession } func makeRequest(with url: URL, completion: @escaping (Result<Data, Error>) -> Void) { let task = urlSession.dataTask(with: url) { data, response, error in // Handle network response } task.resume() } }
In this example, we have defined a protocol URLSessionProtocol
that defines the methods required for network requests. We then extend the URLSession
class to conform to this protocol.
The NetworkingService
class now depends on the URLSessionProtocol
abstraction, rather than the URLSession
class directly. This allows us to provide a different implementation of the URLSessionProtocol
in the future, without having to modify the NetworkingService
class.
For example, we can create a mock implementation of the URLSessionProtocol
for testing purposes:
class MockURLSession: URLSessionProtocol { func dataTask(with url: URL, completionHandler: @escaping (Data?, URLResponse?, Error?) -> Void) -> URLSessionDataTask { // Return mock data task for testing purposes } }
And we can use this mock implementation to test the NetworkingService
class:
func testNetworkingService() { let mockUrlSession = MockURLSession() let networkingService = NetworkingService(urlSession: mockUrlSession) // Perform networking request and assert results }
In this way, we have applied the DIP by depending on abstractions rather than low-level modules, and we can easily swap out different implementations of the URLSessionProtocol
without affecting the functionality of the NetworkingService
class.
POP
You can enforce some common SOLID principles by adapting POP to iOS. Let me explain –
Protocol Oriented Programming (POP) is a programming paradigm that emphasizes the use of protocols to define interfaces and behavior. Instead of focusing on creating classes and inheritance hierarchies, POP emphasizes defining protocols that describe the behavior that types should conform to.
In iOS programming, POP is particularly powerful because it allows for greater flexibility and modularity in code. By defining protocols that describe the behavior of types, it becomes easier to compose and reuse code across different parts of an application. This is particularly important in iOS development, where code reuse is often a key goal due to the platform’s constraints on memory usage and processing power.
POP also allows for more efficient code, since protocols can be used to define behavior that can be implemented by multiple types, rather than requiring redundant code to be written for each individual type. Additionally, POP can help to reduce the complexity of code, since it encourages developers to break down behavior into smaller, more manageable pieces that can be more easily tested and maintained. POP is particularly well-suited to Swift, the programming language used for iOS development, since Swift was designed with a focus on protocol-oriented programming. Swift’s protocol-oriented features, such as protocol extensions and protocol inheritance, make it particularly easy to define and work with protocols in code. Protocol Oriented Programming (POP) can help enforce the SOLID principles in iOS code bases in the following ways:
- Single Responsibility Principle (SRP): POP encourages developers to define protocols that describe a single behavior or responsibility, making it easier to design types that adhere to the SRP. By breaking down behavior into smaller, more focused protocols, developers can ensure that each type has a clear and specific responsibility.
- Open/Closed Principle (OCP): POP allows developers to define protocols that can be extended by conforming types, rather than relying on inheritance hierarchies. This makes it easier to add new behavior to an application without modifying existing code, and helps ensure that types remain closed to modification but open to extension.
- Liskov Substitution Principle (LSP): Since protocols define behavior rather than implementation details, conforming types can be easily substituted for one another without causing issues. This makes it easier to compose and reuse code across an application without introducing unexpected behavior.
- Interface Segregation Principle (ISP): POP encourages the use of protocols to define interfaces, which makes it easier to define smaller, more focused interfaces that are tailored to specific needs. This helps ensure that types only depend on the behavior they need, and reduces the likelihood of dependencies on unnecessary behavior.
- Dependency Inversion Principle (DIP): By defining protocols that describe behavior rather than concrete types, POP makes it easier to design types that depend on abstractions rather than concretions. This can make code more flexible and easier to test, since dependencies can be easily mocked or substituted with different implementations.
My Personal Finding while Mentoring- SOLID for Junior iOS Developers
Applying SOLID principles can be challenging for junior iOS developers who are just starting out in app development. SOLID principles are a set of guidelines for writing clean, maintainable, and scalable code, and they require a good understanding of object-oriented programming concepts, POP, and design patterns. Junior developers who are new to iOS development may not have had much experience working with complex codebases, and may struggle to apply SOLID principles in a practical context. Additionally, SOLID principles can be difficult to understand and implement without guidance from more experienced developers.
However, this does not mean that junior developers cannot learn and apply SOLID principles. With proper guidance and practice, junior developers can develop a strong understanding of SOLID principles and apply them effectively in their iOS development work. Providing feedback and code reviews can help junior developers improve their understanding of SOLID principles and develop their coding skills over time.
Thanks for reading.
✍️ Written by Ishtiak Ahmed
👉 Follow me on X ● LinkedIn
Get Ready to Shine: Mastering the iOS Interview
Enjoying the articles? Get the inside scoop by subscribing to my newsletter.
Get access to exclusive iOS development tips, tricks, and insights when you subscribe to my newsletter. You'll also receive links to new articles, app development ideas, and an interview preparation mini book.