Uplift iOS Interview
The Guide is for YOU if
- You are preparing for an iOS interview and want to improve your skills and knowledge and looking to level up your interview game and land your dream job.
- You want to gain confidence and ease during iOS interviews by learning expert tips and curated strategies.
- You want access to a comprehensive list of iOS interview QA to practice and prepare.
Swift is a powerful programming language that has become the go-to choice for iOS developers since its release in 2014. Swift has a clean syntax, offers better performance than its predecessor Objective-C, and allows for safer coding with its strong typing system. If you’re an iOS developer, there’s a good chance you’re already familiar with Swift. In this blog post, we’ll take a look at some curated tips for Swift in iOS development and the benefits they bring.
Use Optionals
One of the most powerful features of Swift is optionals. Optionals allow for a variable or constant to contain either a value or nil. This is particularly useful in iOS development because it allows for more robust handling of data. When working with APIs, for example, you may receive data that is missing a particular field. Using optionals allows you to handle this situation without crashing your app.
Guard and if let statements
Guard and if let statements are powerful tools for handling optionals. Guard statements allow for early exits from a function if a condition isn’t met. If let statements allow for safely unwrapping an optional and using its value if it’s not nil. Both of these statements can help reduce code complexity and improve the safety of your app.
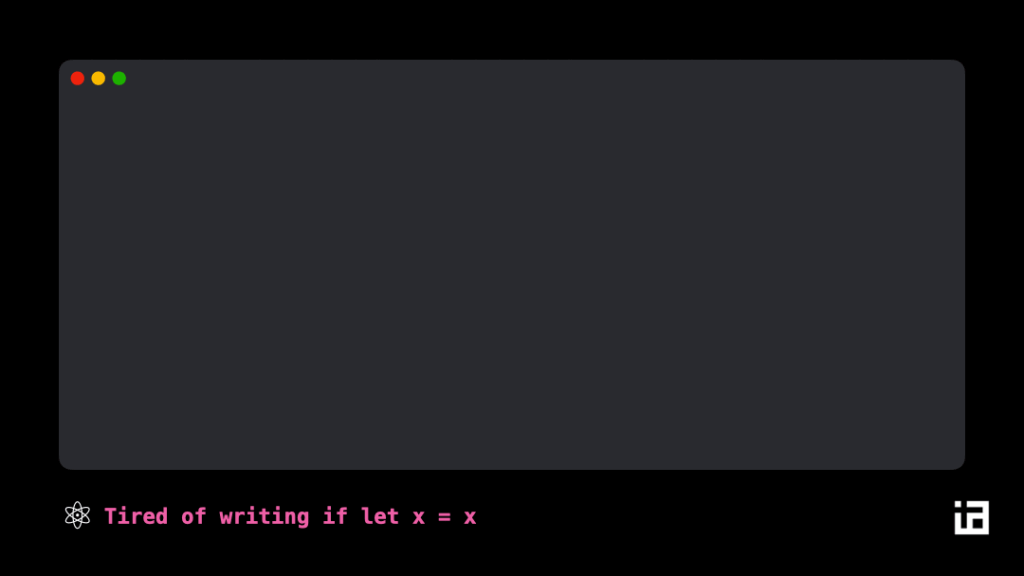
Use Enums for State
Enums can be used to define the state of your app. This can include the state of a network request, the state of a view controller, or the state of a user’s authentication status. Using enums for state helps to make your code more readable and easier to maintain. It also helps to ensure that your code is handling all possible states.
Use Extensions to Add Functionality
Extensions allow for adding functionality to existing classes or structs. This is particularly useful in iOS development because it allows for customizing UI elements. For example, you could use an extension to add a border or corner radius to a button. Extensions can also be used to add functionality to built-in classes, such as adding a custom method to the String class.
Protocol-oriented programming
Protocol-oriented programming is a powerful concept in Swift. It allows for defining protocols that describe a set of behaviors. Classes and structs can then conform to these protocols, which allows for more generic programming. This is particularly useful in iOS development because it allows for more flexible and reusable code.
Long Numbers
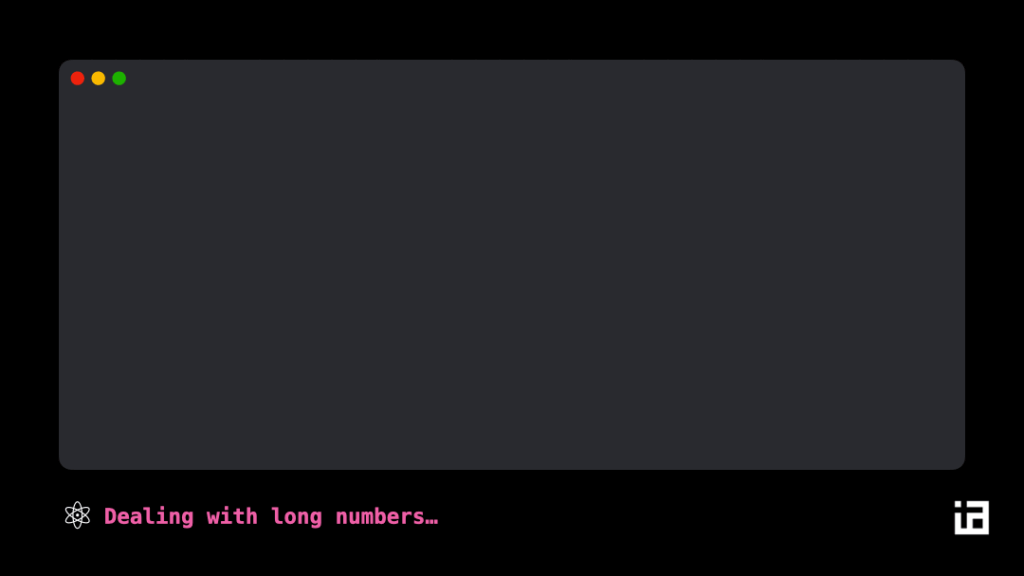
Use Descriptive Names
Using descriptive and meaningful names for variables, functions, and classes is essential for writing clean and understandable code. When naming, consider the purpose and functionality of the variable, function, or class.
Follow Code Conventions
Adhering to code conventions and style guides can improve the readability of your code. Apple provides an official Swift style guide that you can follow, but you can also create your own style guide for your team.
Avoid Force Unwrapping/Avoid Using Implicitly Unwrapped Optionals
Force unwrapping an optional can lead to runtime errors if the optional is nil. It’s better to use optional binding or optional chaining to safely unwrap optionals.
Use Structs When Appropriate
In Swift, structs are value types that can be copied and mutated without affecting the original instance. Structs are a good choice when you need a lightweight data type or when you want to create a snapshot of an object.
Avoid Global Variables
Global variables can make your code harder to understand and maintain. Instead, consider using dependency injection or a singleton pattern to share data between objects.
Test Your Code
Writing tests can help you catch bugs and ensure that your code is functioning as expected. There are several testing frameworks available for Swift, including XCTest and Quick.
This page is continuously updated with new tips. I highly recommend bookmarking it or joining my Newsletter.
In conclusion, Swift is a powerful language for iOS development, and by using these curated tips, you can take your app development to the next level. Whether you’re a seasoned iOS developer or just starting out, these tips can help improve the quality of your code and make your app development experience smoother and more efficient.
✍️ Written by Ishtiak Ahmed
👉 Follow me on X ● LinkedIn
Get Ready to Shine: Mastering the iOS Interview
Enjoying the articles? Get the inside scoop by subscribing to my newsletter.
Get access to exclusive iOS development tips, tricks, and insights when you subscribe to my newsletter. You'll also receive links to new articles, app development ideas, and an interview preparation mini book.